Nov 28, 2015 In this tutorial we’ll be using py2app to create a standalone OSX application from a Python 2 or 3 source code with a simple Tkinter user interface. 'py2app is a Python setuptools command which will allow you to make standalone application bundles and plugins from Python scripts. Py2app is similar in purpose and design to py2exe for Windows.' Relevant links about py2app: Documentation Source.
-->In this 5-10 minute introduction to Visual Studio as a Python IDE, you create a simple Python web application based on the Flask framework. You create the project through discrete steps that help you learn about Visual Studio's basic features.
If you haven't already installed Visual Studio, go to the Visual Studio downloads page to install it for free. In the installer, make sure to select the Python development workload.
If you haven't already installed Visual Studio, go to the Visual Studio downloads page to install it for free. In the installer, make sure to select the Python development workload.
Create the project
The following steps create an empty project that serves as a container for the application:
Open Visual Studio 2017.
From the top menu bar, choose File > New > Project.
In the New Project dialog box, enter 'Python Web Project' in the search field on the upper right, choose Web project in the middle list, give the project a name like 'HelloPython', then choose OK.
If you don't see the Python project templates, run the Visual Studio Installer, select More > Modify, select the Python development workload, then choose Modify.
The new project opens in Solution Explorer in the right pane. The project is empty at this point because it contains no other files.
Open Visual Studio 2019.
On the start screen, select Create a new project.
In the Create a new project dialog box, enter 'Python web' in the search field at the top, choose Web Project in the middle list, then select Next:
If you don't see the Python project templates, run the Visual Studio Installer, select More > Modify, select the Python development workload, then choose Modify.
In the Configure your new project dialog that follows, enter 'HelloPython' for Project name, specify a location, and select Create. (The Solution name is automatically set to match the Project name.)
The new project opens in Solution Explorer in the right pane. The project is empty at this point because it contains no other files.
Question: What's the advantage of creating a project in Visual Studio for a Python application?
Answer: Python applications are typically defined using only folders and files, but this simple structure can become burdensome as applications become larger and perhaps involve auto-generated files, JavaScript for web applications, and so on. A Visual Studio project helps manage this complexity. The project (a .pyproj file) identifies all the source and content files associated with your project, contains build information for each file, maintains the information to integrate with source-control systems, and helps you organize your application into logical components.
Question: What is the 'solution' shown in Solution Explorer?
Answer: A Visual Studio solution is a container that helps you manage for one or more related projects as a group, and stores configuration settings that aren't specific to a project. Projects in a solution can also reference one another, such that running one project (a Python app) automatically builds a second project (such as a C++ extension used in the Python app).
Install the Flask library
Web apps in Python almost always use one of the many available Python libraries to handle low-level details like routing web requests and shaping responses. For this purpose, Visual Studio provides a variety of templates for web apps, one of which you use later in this Quickstart.
Here, you use the following steps to install the Flask library into the default 'global environment' that Visual Studio uses for this project.
Expand the Python Environments node in the project to see the default environment for the project.
Right-click the environment and select Install Python Package. This command opens the Python Environments window on the Packages tab.
Description:This file contains the EpsonNet Config Utility v4.9.5.EpsonNet Config is a configuration utility for administrators to configure the network interface. For a portable version of this file, please download.Compatible systems:Windows 10 32-bit, Windows 10 64-bit, Windows 8.1 32-bit, Windows 8.1 64-bit, Windows 8 32-bit, Windows 8 64-bit, Windows 7 32-bit, Windows 7 64-bit, Windows XP 32-bit, Windows XP 64-bit, Windows Vista 32-bit, Windows Vista 64-bitNote:This file applies to numerous Epson products and may be compatible with operating systems that your model is not. Epson software download. Description:This file contains the EpsonNet Config Utility v4.9.5.EpsonNet Config is a configuration utility for administrators to configure the network interface.
Enter 'flask' in the search field and select pip install flask from PyPI. Accept any prompts for administrator privileges and observe the Output window in Visual Studio for progress. (A prompt for elevation happens when the packages folder for the global environment is located within a protected area like C:Program Files.)
Expand the Python Environments node in the project to see the default environment for the project.
Right-click the environment and select Manage Python Packages... This command opens the Python Environments window on the Packages (PyPI) tab.
Enter 'flask' in the search field. If Flask appears below the search box, you can skip this step. Otherwise select Run command: pip install flask. Accept any prompts for administrator privileges and observe the Output window in Visual Studio for progress. (A prompt for elevation happens when the packages folder for the global environment is located within a protected area like C:Program Files.)
Once installed, the library appears in the environment in Solution Explorer, which means that you can make use of it in Python code.
Note
Instead of installing libraries in the global environment, developers typically create a 'virtual environment' in which to install libraries for a specific project. Visual Studio templates typically offer this option, as discussed in Quickstart - Create a Python project using a template.
Question: Where do I learn more about other available Python packages?
Answer: Visit the Python Package Index.
Add a code file
You're now ready to add a bit of Python code to implement a minimal web app.
Right-click the project in Solution Explorer and select Add > New Item.
In the dialog that appears, select Empty Python File, name it app.py, and select Add. Visual Studio automatically opens the file in an editor window.
Copy the following code and paste it into app.py:
You may have noticed that the Add > New Item dialog box displays many other types of files you can add to a Python project, including a Python class, a Python package, a Python unit test, web.config files, and more. In general, these item templates, as they're called, are a great way to quickly create files with useful boilerplate code.
Question: Where can I learn more about Flask?
I messenger app for mac. ,536000000,2911,null,null,'5','Barbu Kovu00e1cs',null,null,2,null,null,null,'shinthantoo',null,2,null,null,null,'app is really great to use. Just one note, I think it is a shame that a large company like Facebook does so little for their users, yet it is still the largest database of people, so you cannot really delete the whole thing all together.' I always make video chat on it. I do not really know if this has something to do with the recent android system update, but these apps should adapt to it too, surely.
Answer: Refer to the Flask documentation, starting with the Flask Quickstart.
Run the application
Right-click app.py in Solution Explorer and select Set as startup file. This command identifies the code file to launch in Python when running the app.
Right-click the project in Solution Explorer and select Properties. Then select the Debug tab and set the Port Number property to
4449
. This step ensures that Visual Studio launches a browser withlocalhost:4449
to match theapp.run
arguments in the code.Select Debug > Start Without Debugging (Ctrl+F5), which saves changes to files and runs the app.
A command window appears with the message Running in https://localhost:4449, and a browser window should open to
localhost:4449
where you see the message, 'Hello, Python!' The GET request also appears in the command window with a status of 200.If a browser does not open automatically, start the browser of your choice and navigate to
localhost:4449
.If you see only the Python interactive shell in the command window, or if that window flashes on the screen briefly, ensure that you set app.py as the startup file in step 1 above.
Navigate to
localhost:4449/hello
to test that the decorator for the/hello
resource also works. Again, the GET request appears in the command window with a status of 200. Feel free to try some other URL as well to see that they show 404 status codes in the command window.Close the command window to stop the app, then close the browser window.
Question: What's the difference between the Start Without Debugging command and Start Debugging?
Answer: You use Start Debugging to run the app in the context of the Visual Studio debugger, allowing you to set breakpoints, examine variables, and step through your code line by line. Apps may run slower in the debugger because of the various hooks that make debugging possible. Start Without Debugging, in contrast, runs the app directly as if you ran it from the command line, with no debugging context, and also automatically launches a browser and navigates to the URL specified in the project properties' Debug tab.
Next steps
Congratulations on running your first Python app from Visual Studio, in which you've learned a little about using Visual Studio as a Python IDE!
Because the steps you followed in this Quickstart are fairly generic, you've probably guessed that they can and should be automated. Such automation is the role of Visual Studio project templates. Go through Quickstart - Create a Python project using a template for a demonstration that creates a web app similar to the one you created in this article, but with fewer steps.
To continue with a fuller tutorial on Python in Visual Studio, including using the interactive window, debugging, data visualization, and working with Git, go through Tutorial: Get started with Python in Visual Studio.
To explore more that Visual Studio has to offer, select the links below.
- Learn about Python web app templates in Visual Studio.
- Learn about Python debugging
- Learn more about the Visual Studio IDE in general.
Azure Pipelines
Use a pipeline to automatically build and test your Python apps or scripts. After those steps are done, you can then deploy or publish your project.
If you want an end-to-end walkthrough, see Use CI/CD to deploy a Python web app to Azure App Service on Linux.
To create and activate an Anaconda environment and install Anaconda packages with conda
, see Run pipelines with Anaconda environments.
Create your first pipeline
Are you new to Azure Pipelines? If so, then we recommend you try this section before moving on to other sections.
Get the code
Import this repo into your Git repo in Azure DevOps Server 2019:
Sign in to Azure Pipelines
Sign in to Azure Pipelines. After you sign in, your browser goes to https://dev.azure.com/my-organization-name
and displays your Azure DevOps dashboard.
Within your selected organization, create a project. If you don't have any projects in your organization, you see a Create a project to get started screen. Otherwise, select the Create Project button in the upper-right corner of the dashboard.
Create the pipeline
Sign in to your Azure DevOps organization and navigate to your project.
Go to Pipelines, and then select New Pipeline.
Walk through the steps of the wizard by first selecting GitHub as the location of your source code.
You might be redirected to GitHub to sign in. If so, enter your GitHub credentials.
When the list of repositories appears, select your repository.
You might be redirected to GitHub to install the Azure Pipelines app. If so, select Approve and install.
When the Configure tab appears, select Python package. This will create a Python package to test on multiple Python versions.
When your new pipeline appears, take a look at the YAML to see what it does. When you're ready, select Save and run.
You're prompted to commit a new azure-pipelines.yml file to your repository. After you're happy with the message, select Save and run again.
If you want to watch your pipeline in action, select the build job.
You just created and ran a pipeline that we automatically created for you, because your code appeared to be a good match for the Python package template.
You now have a working YAML pipeline (
azure-pipelines.yml
) in your repository that's ready for you to customize!When you're ready to make changes to your pipeline, select it in the Pipelines page, and then Edit the
azure-pipelines.yml
file.
See the sections below to learn some of the more common ways to customize your pipeline.
YAML
- Add an
azure-pipelines.yml
file in your repository. Customize this snippet for your build.
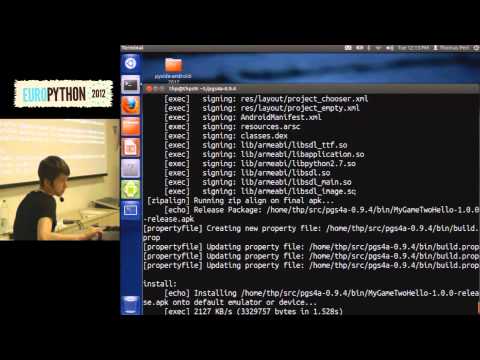
Create a pipeline (if you don't know how, see Create your first pipeline), and for the template select YAML.
Set the Agent pool and YAML file path for your pipeline.
Save the pipeline and queue a build. When the Build #nnnnnnnn.n has been queued message appears, select the number link to see your pipeline in action.
When you're ready to make changes to your pipeline, Edit it.
See the sections below to learn some of the more common ways to customize your pipeline.
Build environment
You don't have to set up anything for Azure Pipelines to build Python projects. Python is preinstalled on Microsoft-hosted build agents for Linux, macOS, or Windows. To see which Python versions are preinstalled, see Use a Microsoft-hosted agent.
Use a specific Python version
To use a specific version of Python in your pipeline, add the Use Python Version task to azure-pipelines.yml. This snippet sets the pipeline to use Python 3.6:
Use multiple Python versions
To run a pipeline with multiple Python versions, for example to test a package against those versions, define a job
with a matrix
of Python versions. Then set the UsePythonVersion
task to reference the matrix
variable.
You can add tasks to run using each Python version in the matrix.
Run Python scripts
To run Python scripts in your repository, use a script
element and specify a filename. For example:
You can also run inline Python scripts with the Python Script task:
To parameterize script execution, use the PythonScript
task with arguments
values to pass arguments into the executing process. You can use sys.argv
or the more sophisticated argparse
library to parse the arguments.
Install dependencies
You can use scripts to install specific PyPI packages with pip
. For example, this YAML installs or upgrades pip
and the setuptools
and wheel
packages.
Install requirements
After you update pip
and friends, a typical next step is to install dependencies from requirements.txt:
Run tests
You can use scripts to install and run various tests in your pipeline.
Run lint tests with flake8
To install or upgrade flake8
and use it to run lint tests, use this YAML:
Test with pytest and collect coverage metrics with pytest-cov
Use this YAML to install pytest
and pytest-cov
, run tests, output test results in JUnit format, and output code coverage results in Cobertura XML format:
Run tests with Tox
Azure Pipelines can run parallel Tox test jobs to split up the work. On a development computer, you have to run your test environments in series. This sample uses tox -e py
to run whichever version of Python is active for the current job.
Publish test results
Add the Publish Test Results task to publish JUnit or xUnit test results to the server:
Publish code coverage results
Add the Publish Code Coverage Results task to publish code coverage results to the server. You can see coverage metrics in the build summary, and download HTML reports for further analysis.
Package and deliver code
Build Mac App With Python Download
To authenticate with twine
, use the Twine Authenticate task to store authentication credentials in the PYPIRC_PATH
environment variable.
Create Android App With Python
Then, add a custom script that uses twine
to publish your packages.
You can also use Azure Pipelines to build an image for your Python app and push it to a container registry.
Related extensions

Run Python On Mac
- PyLint Checker (Darren Fuller)
- Python Test (Darren Fuller)
- Azure DevOps plugin for PyCharm (IntelliJ) (Microsoft)
- Python in Visual Studio Code (Microsoft)